Basic Cellular Network Analytics¶
The following examples give a quick overview of the MobileInsight API.
Contents
Conceptual Overview¶
The MobileInsight core has two types of modules: monitors and analyzers. The monitors collect raw cellular logs and parse them into protocol messages. These messages would further drive the mobile network analysis. The analyzers are event-driven, and can perform online/offline mobile network analysis.
The MobileInsight analysis framework is modular and extensible by design. To perform a cellular monitoring/analysis task, the user needs to declare one monitor module and any numbers of analyzer modules, connect the analyzers to the monitor, and then start the analysis. If necessary, the users can write their own analyzers for specific processing purpose. To facilitate code reuse, the users can build their own analyzers on top of existing ones.
A “Hello World” Task: Monitoring Cellular Logs¶
We start from the simplest task: monitoring cellular logs only. The following code shows how to collect cellular logs and save them to disk (also available in mobile_insight/examples/monitor-example.py in the desktop version, which can be executed on both desktop server and phone):
import os
import sys
# Import MobileInsight modules
from mobile_insight.monitor import OnlineMonitor
from mobile_insight.analyzer import MsgLogger
if len(sys.argv) < 3:
opt.error("please specify physical port name and baudrate.")
sys.exit(1)
# Initialize a 3G/4G/5G monitor
src = OnlineMonitor()
src.set_serial_port(sys.argv[1]) #the serial port to collect the traces
src.set_baudrate(int(sys.argv[2])) #the baudrate of the port
# Specify logs to be collected: RRC (radio resource control) in this example
src.enable_log("5G_NR_RRC_OTA_Packet") # 5G RRC
src.enable_log("LTE_RRC_OTA_Packet") # 4G LTE RRC
src.enable_log("WCDMA_RRC_OTA_Packet") # 3G WCDMA RRC
# Save the monitoring results as an offline log
src.save_log_as("./monitor-example.mi2log")
# Start the monitoring
src.run()
In this code, we declare a OnlineMonitor to monitor 3G/4G cellular network messages. To specifiy the cellular logs to be collected, the enable_log( ) method should be called with specific log types (more supported messages can be found here). No analyzers are declared since this code does not perform any analysis task. When this code is executed, the cellular logs will be saved to monitor-example.mi2log, which can be either used for offline analysis (details below) or opened with MobileInsight GUI.
Single Analysis Task: Printing Cellular Logs¶
We next show how to perform analysis tasks. We extend above code with a simple analysis task: print out the cellular logs on the fly. To achieve this, we declare a bulit-in analyzer MsgLogger, which can decode each message into XML format (JSON is also supported, see here for more details). Then we bind it to the monitor. At runtime, the monitor will pushes the cellular logs to the analyzer, which further performs specific analysis:
import os
import sys
# Import MobileInsight modules
from mobile_insight.monitor import OnlineMonitor
from mobile_insight.analyzer import MsgLogger
if len(sys.argv) < 3:
opt.error("please specify physical port name and baudrate.")
sys.exit(1)
# Initialize a 3G/4G monitor
src = OnlineMonitor()
src.set_serial_port(sys.argv[1]) #the serial port to collect the traces
src.set_baudrate(int(sys.argv[2])) #the baudrate of the port
# Specify logs to be collected: RRC (radio resource control) in this example
src.enable_log("5G_NR_RRC_OTA_Packet") # 5G RRC
src.enable_log("LTE_RRC_OTA_Packet") # 4G LTE RRC
src.enable_log("WCDMA_RRC_OTA_Packet") # 3G WCDMA RRC
# Save the monitoring results as an offline log
src.save_log_as("./monitor-example.mi2log")
#Print messages.
dumper = MsgLogger() #Declare an analyzer
dumper.set_source(src) #Bind the analyzer to the monitor
dumper.set_decoding(MsgLogger.XML) #decode the message as xml
# Start the monitoring
src.run()
Composable Analysis¶
MobileInsight supports modular analysis: multiple analyzers can be connected to the single monitor. This helps to build more complex analysis tasks. As an example, the following code monitors the 3G/4G RRC, and reports the current cell status with WcdmaRrcAnalyzer and LteRrcAnalyzer (also available in mobile_insight/examples/online-analysis-example.py):
import os
import sys
from mobile_insight.monitor import OnlineMonitor
from mobile_insight.analyzer import LteRrcAnalyzer,WcdmaRrcAnalyzer
if len(sys.argv) < 3:
opt.error("please specify physical port name and baudrate.")
sys.exit(1)
# Initialize a monitor
src = OnlineMonitor()
src.set_serial_port(sys.argv[1]) #the serial port to collect the traces
src.set_baudrate(int(sys.argv[2])) #the baudrate of the port
# 5G RRC analyzer
nr_rrc_analyzer = NrRrcAnalyzer()
nr_rrc_analyzer.set_source(src) # bind with the monitor
# 4G RRC analyzer
lte_rrc_analyzer = LteRrcAnalyzer()
lte_rrc_analyzer.set_source(src) #bind with the monitor
# 3G RRC analyzer
wcdma_rrc_analyzer = WcdmaRrcAnalyzer()
wcdma_rrc_analyzer.set_source(src) #bind with the monitor
src.run()
Note that, both LteRrcAnalyzer and WcdmaRrcAnalyzer will automatically enable the corresponding logs at initialization, so there is no need to manually call enable_log( ).
Offline Analysis¶
All above examples are online analysis: they collect cellular logs at runtime and performs analysis. Besides, MobileInsight also supports offline analysis: you can load a saved log (in *.mi2log format) and drive the analysis. This is useful for several cases. For example, some complex analysis would be better performed offline, such as batch processing. Besides, offline analysis can also be used for replay-based debugging (e.g., when developing your own analyzers).
MobileInsight supports two ways of offline analysis: you can either manually analyze the *.mi2log log with MobileInsight GUI, or programmatically analyze the logs with OfflineReplayer, a special type of monitor. The following example shows how to use OfflineReplayer for the offline analysis (also available in mobile_insight/examples/offline-analysis-example.py):
import os
import sys
from mobile_insight.monitor import OfflineReplayer
from mobile_insight.analyzer import LteRrcAnalyzer,WcdmaRrcAnalyzer
src = OfflineReplayer()
# Load offline logs
src.set_input_path("./offline_log_examples/")
# RRC analyzer
nr_rrc_analyzer = NrRrcAnalyzer() # 5G NR
nr_rrc_analyzer.set_source(src) # bind with the monitor
lte_rrc_analyzer = LteRrcAnalyzer() # 4G LTE
lte_rrc_analyzer.set_source(src) #bind with the monitor
wcdma_rrc_analyzer = WcdmaRrcAnalyzer() # 3G WCDMA
wcdma_rrc_analyzer.set_source(src) #bind with the monitor
src.run()
The analysis results would be shown as follows.
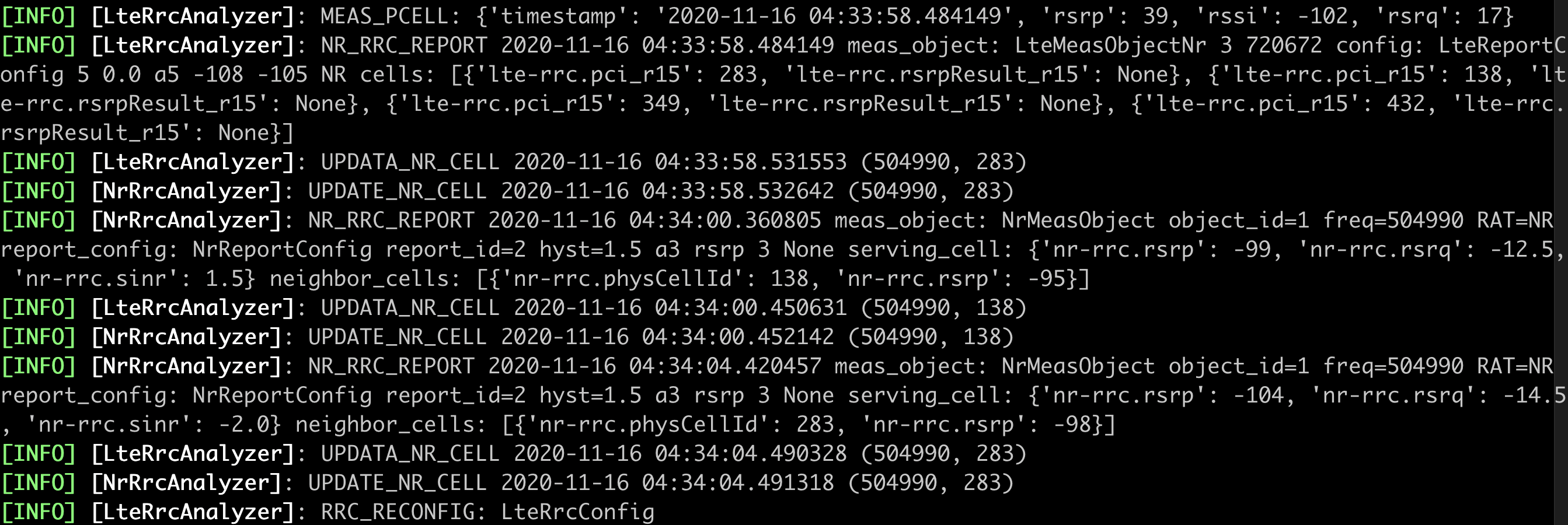
Built-in Analyzers¶
To facilitate analysis, MobileInsight has provided several built-in analyzers. Currently it provides analyzers to track major 3G/4G protocols, including the radio resource control (RRC), mobility management (MM/GMM/EMM), session management (CM/SM/ESM), physical layer, etc. More analyzers will be provided in the future versions.